Roblox Studio is a powerful tool for creating games, but many beginners make simple mistakes that slow them down. These mistakes can cause errors, break your game, or make development frustrating. Learning to avoid them will save you time and improve your skills.
1. Not Organizing the Explorer Panel
When you add parts, scripts, and models, they appear in the Explorer panel. If you don’t organize them, your game will definitely become messy, and will create a very annoying environment to deal with when trying to develop your game. You’ll waste lots of valuable time chasing imaginary objects and parts, and troubleshooting the most random errors.
Solution:
- Use folders to group items that are relatively close to each other either in terms of distance, or in function.
- Rename parts instead of keeping “Part1” or “Model2.” This is programming 101! If you don’t rename a part or object, eventually, you’ll have to run through your entire game just to find it!
A clean explorer tab will further streamline and allow for a much more convenient developing experience.
2. Forgetting to Anchor Parts
If a part isn’t anchored, it will fall due to gravity when the game starts. Many beginners build structures and wonder why they suddenly fall apart like a tower of playing cards.
Fix:
- Select a part, then check the “Anchor” box in the Properties panel. Doesn’t get easier than that!
- Use “Anchor All” to quickly fix multiple parts. Personally, I would not recommend anchoring all parts in your game, as obviously some will need to freely move, unless your game is completely stationary (boring!)
But either way, anything that should stay in place—like buildings, platforms, or NPCs—must be anchored. Imagine Big Ben falling towards your head at top speeds! Not fun I must say.
3. Free Models
The Toolbox lets you insert Free Models, but some contain bad scripts. These scripts may spam your game, steal data, or cause lag. Rookie mistake.
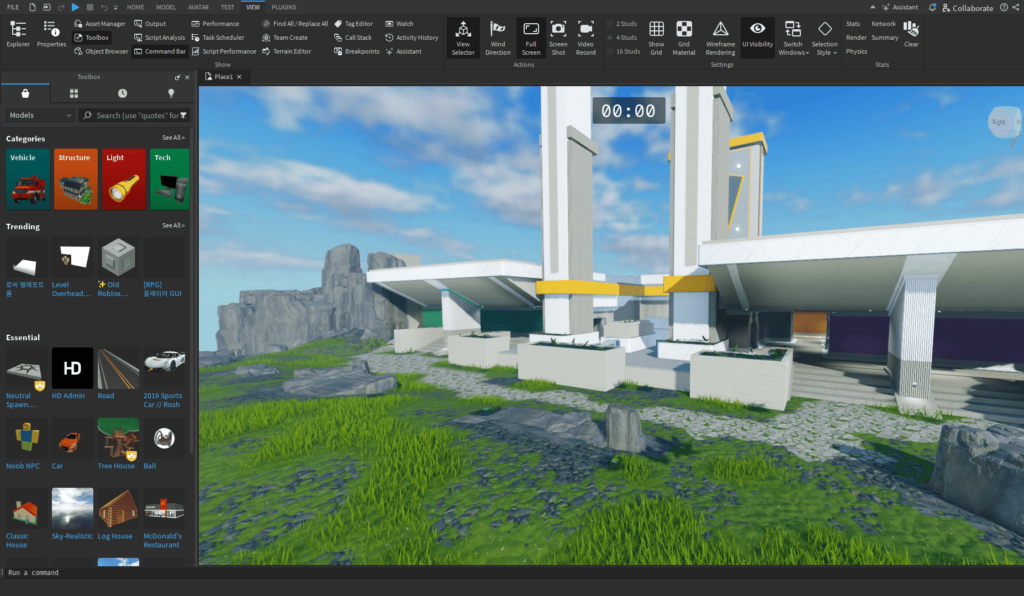
Solution to this:
- Check scripts inside every Free Model before using them. There are many users who maliciously sneak in viruses and unwanted scripts that can harm the user experience in your game.
- Delete unknown scripts, especially those with “Require()” or “InsertService()”. These functions are usually associated with backdoors and other malicious pieces of code
- Use models from trusted creators or make your own. A good route to go down is searching for models by the official ROBLOX account!
Free Models can save time, but more often than not, they’re not worth it.
4. Ignoring the Output Panel
When something breaks, the Output panel tells you why. Many beginners don’t open it, making debugging harder.
Instructions:
- Open View > Output in Roblox Studio.
- When a script fails, read the error message.
- Click the error to jump to the problem in your script.
Debugging your code is a MASSIVE part of game development, and getting this simple habit down is always a good start.
5. Using Too Many Scripts
Some beginners create a separate script for everything. This can cause lag, and make the explorer (as mentioned before) extremely disorganised
How to Fix It:
- Group related code into fewer scripts.
- Use functions to avoid repeating code.
- Learn ModuleScripts to share code between scripts.
Fewer, well-organized scripts keep your game running smoothly.
6. Using the Wrong Script Type for UI
Normal scripts don’t work well for UI elements like buttons or menus. Beginners often use the wrong type and wonder why nothing happens.
How to Fix It:
- Use LocalScripts for UI elements.
- Place them in StarterGui or StarterPlayerScripts.
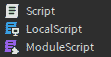
If your button or menu isn’t working, check the script type. Another easy-to-fix issue that can be solved with a quick google search!
7. Not Testing in Multiplayer Mode
Roblox games are often multiplayer, but beginners only test in single-player mode. This causes bugs that only appear with multiple players.
How to Fix It:
- Click Test > Start Server in Studio to run multiplayer tests.
- Use Remote Events to handle player actions properly.
Multiplayer bugs can ruin a game. Test with multiple players early to catch bugs and other potential problems that may arise when playing with more than one player.
8. Not Saving Player Data
If your game has currency, levels, or progress, players expect it to save. Without DataStores, all progress disappears when they leave. That’s like playing a Minecraft world that deletes itself everytime you leave!
Fix:
- Use DataStoreService to save and load player data.
- Here’s a simple example:
local DataStoreService = game:GetService("DataStoreService")
local playerData = DataStoreService:GetDataStore("PlayerData")
game.Players.PlayerAdded:Connect(function(player)
local data = playerData:GetAsync(player.UserId) or {Coins = 0}
player:SetAttribute("Coins", data.Coins)
end)
game.Players.PlayerRemoving:Connect(function(player)
playerData:SetAsync(player.UserId, {Coins = player:GetAttribute("Coins")})
end)
Without DataStores, players will lose everything each time they leave.
9. Overusing While Loops
Loops are useful, but too many can slow down or crash your game. Some beginners use endless while loops when they aren’t needed.
How to Fix It:
Instead of this inefficient code:
while true do
print("Running...")
end
Use RunService, which is better for performance:
game:GetService("RunService").Stepped:Connect(function()
print("Running...")
end)
Use loops only when needed. Too many can cause lag.
10. Copy-Pasting Code Without Learning
Some beginners copy scripts from the internet without understanding them. This leads to errors they can’t fix.
Solution:
- Learn basic Lua scripting instead of relying on others’ code. That’s the most I can recommend. Learn the language!
- The Roblox Developer Hub is a GREAT place to start, as it contains many beginner tutorials, help articles and you can even make a post yourself asking for more support!
Understanding your own code makes you a better developer.